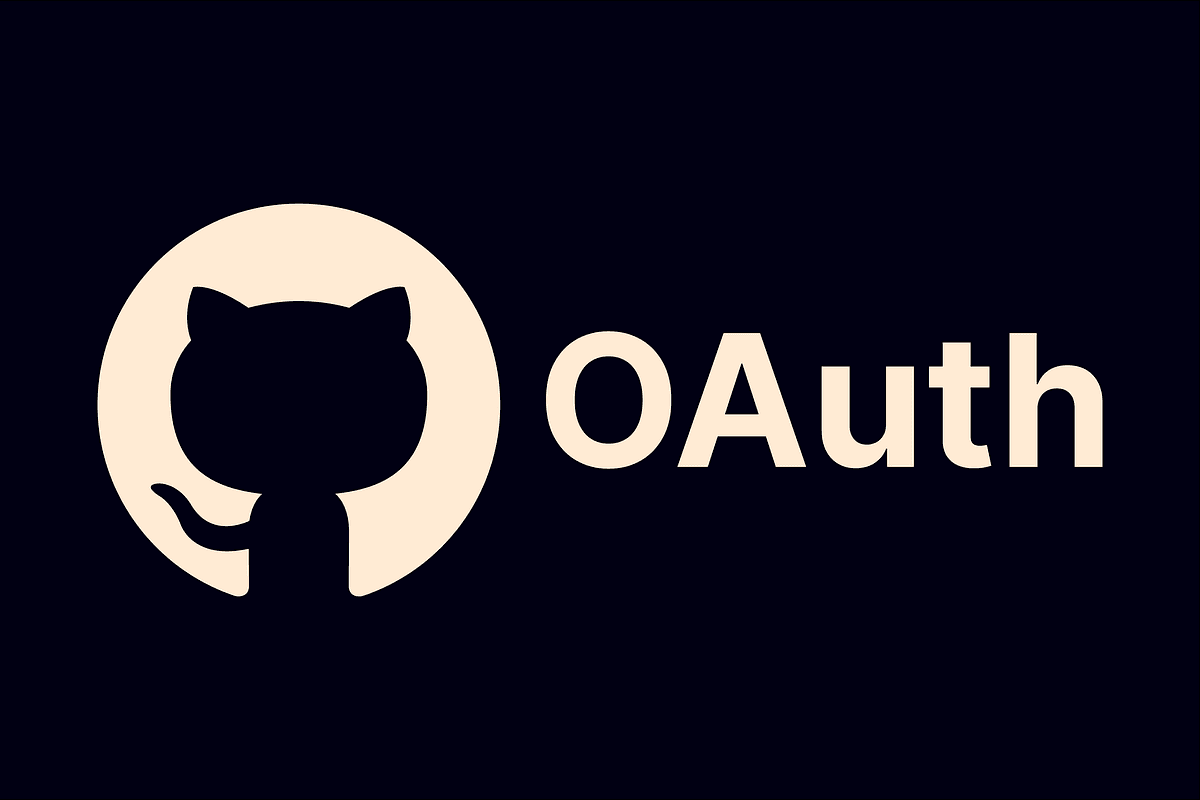
GitHub login authentication with OAuth
14 May 2023

Doris Siu
OAuth is an open standard for authorization that allows users grant third-party applications access to their data without having to share their passwords. It is a more secure way to share information with third-party applications than simply providing your password.
Project
OAuth
OAuth is a widely used standard and is supported by most websites and applications. If you are concerned about the security of your online information, you should consider using OAuth and GitHub also supports OAuth Apps.
Mini demo video
I recorded a short video to go through the part of GitHub login authentication, demonstrated by the project "in-a-class-of-our-own".
Hey! Click me to watch
How OAuth works
Here is a series of steps to grant access to the authorised web application:
1. The user logs in to the service that they want to access.
2. The user is presented with a list of permissions that the application is requesting.
3. The user can then choose to grant/deny these permissions.
4. If the user grants the permissions, the third-party application will be given a token which can be used to access the user's account.
5. The token is only valid for a certain period of time and can be revoked at any time.
Register a new OAuth application
First thing first, you need to set up the OAuth App under your GitHub Account. Here is the path you should follow:
Settings > Developer settings > OAuth App > New Oath App
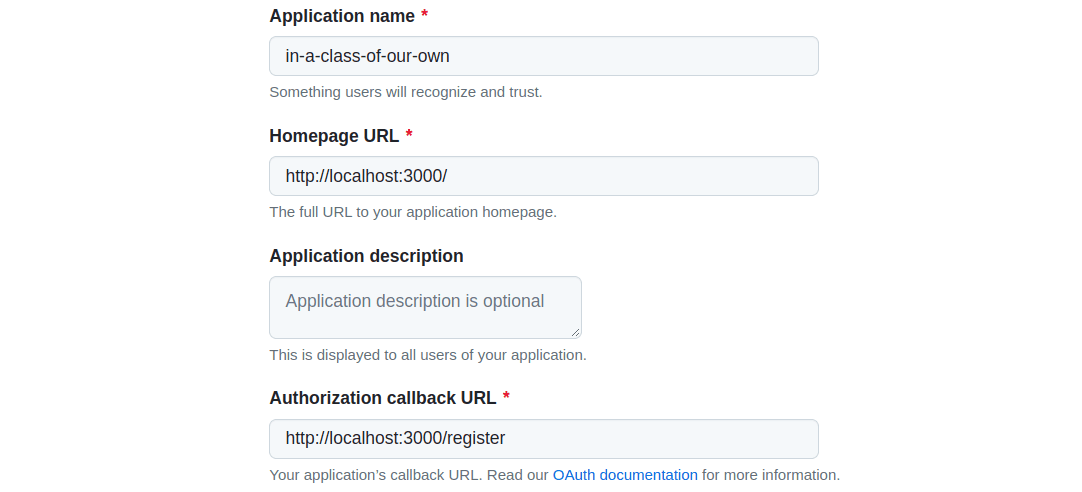
You will see a page like this, where you need to fill your application name, the homepage URL and Authorisation callback URL. After that, you will get a unique Client ID and Client secrets to use in the project.
Token-based identification
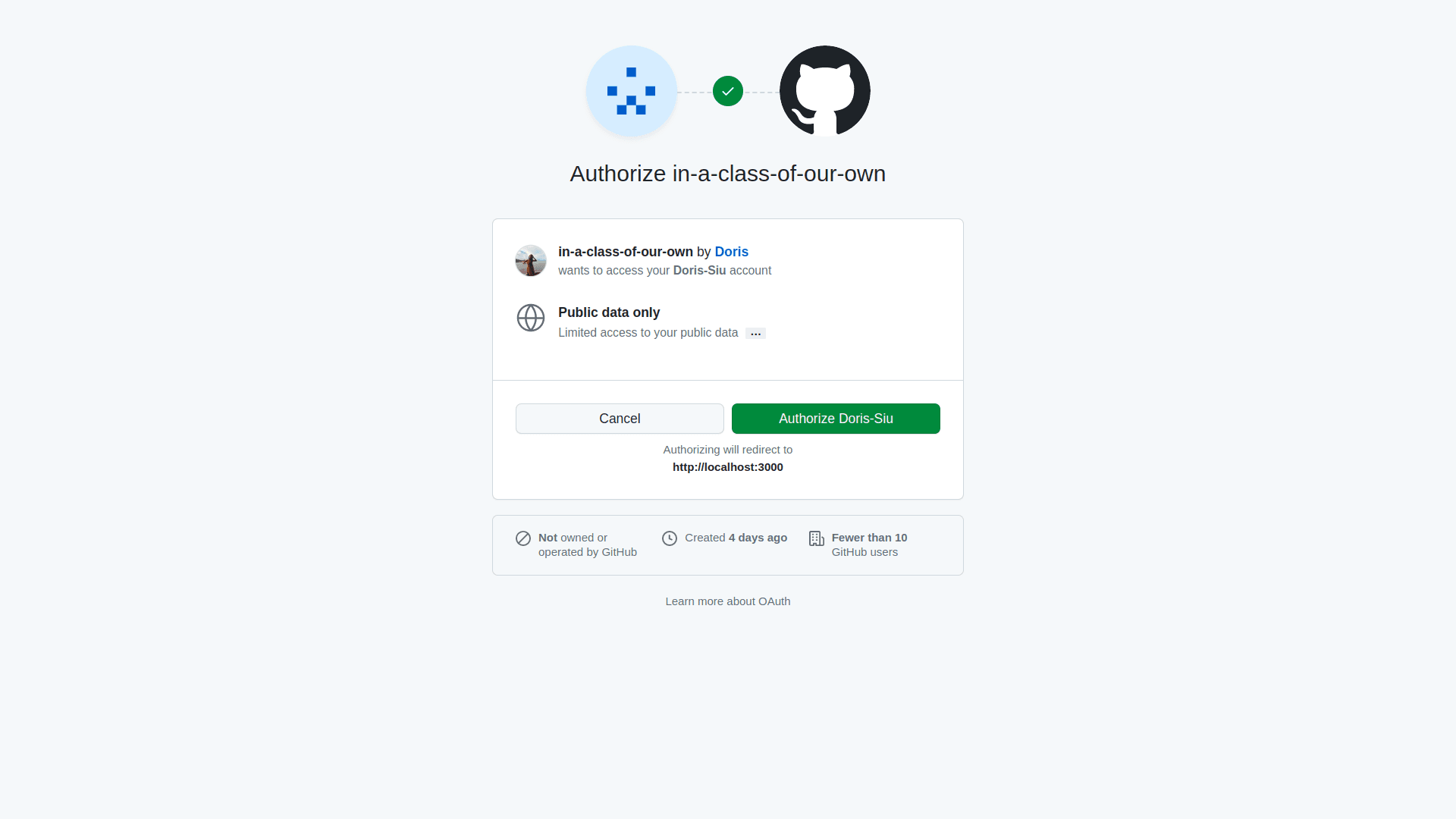
It is a type of authentication that uses a token to verify a user's identity. A token is generated by the server and given to the user. The user then presents the token to the server whenever they want to access the application. Meanwhile, the server can then use the token to verify the user's identity and grant them access to the resource.
If you are looking for a more secure and efficient way to authenticate users, I will recommend token-based identification over traditional password-based authentication. The reason is tokens are not stored on the user's device which cannot be used by reauthorized users. Furthermore, tokens can be easily revoked by one click at anytime.
Here is the code which creates a GET endpoint to get the access token:
router.get("/getAccessToken", async function (req, res) {
const params =
"?client_id=" +
CLIENT_ID +
"&client_secret=" +
CLIENT_SECRET +
"&code=" +
req.query.code;
await fetch("https://github.com/login/oauth/access_token" + params, {
method: "POST",
headers: {
Accept: "application/json",
},
})
.then((response) => response.json())
.then((data) => res.json(data));
});
You can also then pass the returned access token to get the GitHub user data from the following set GET endpoint in the server:
router.get("/getGithubUserData", async function (req, res) {
req.get("Authorization");
await fetch("https://api.github.com/user", {
method: "GET",
headers: {
Authorization: req.get("Authorization"),
},
})
.then((response) => response.json())
.then((data) => res.json(data));
});
From the above endpoints defined in the server side, you can call them flexibly in your client-side application depending whatever you want to do or display.
Insight
To be responsible for this task, it took me quite a lot of time to study and research this brand-new knowledge because I have never experienced any of these kinds of concepts.
However, we learn coding by doing despite the challenge of endless debugging, which somehow frustrates people but I believe that is one of the daily routines of being a developer. The key are the perseverance and curiosity to find the answers. This is undoubtedly a valuable learning outcome to me.
If you want to learn more, check out the codes of the repository here.