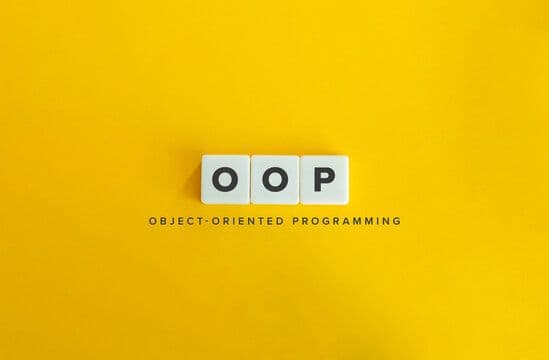
The powerful programming paradigm OOP
30 August 2023

Doris Siu
OOP stands for Object-Oriented Programming which is a powerful programming paradigm used to create complex and well-structured software. It is based on the concept of objects in which the world can be modeled as a collection of objects that interact with each other.
OOP
C#
OOP is one of the fundamental topics in software development and it could be complex for beginners to understand. A simple definition of OOP is that it is based on “objects” which contain fields and methods. A common feature of objects is that methods are attached which can be used to access and modify the object's fields.
However, there are definitely more concepts built around that. In the following, I will give an overview and cover the basics. For your reference, the illustrated codes will be in C#.
Benefits of using OOP:
Increased code reusability:
It allows for code reuse by defining classes that can be used to create new objects, which saves time and effort in the development process.
Increased flexibility:
It allows for more flexibility by enabling objects to behave differently depending on their context which is useful for creating complex software that can adapt to changes.
Increased readability:
It allows for more readability by grouping related data and code together in the same unit in the form of objects. which makes it easier to understand.
Key concepts of OOP:
1. Class & Objects
2. Encapsulation
3. Abstraction
4. Inheritance
5. Polymorphism
Class and objects
To start with, you must be able to understand the idea and difference between class and objects. A class acts as the blueprint for creating objects which defines the data (fields) and code (methods) that all objects will have. It is a collection of objects and is a data type that doesn't consume any space in the memory.
Meanwhile, an object is a self-contained unit that has fields and methods. It is also called an instance of a class and takes some space in memory.
For example, Person is a class and its objects can be Doris, Anna, Billy…
A class “Person” declaration:
public class Person
{
// Define two fields
public string FirstName;
public string LastName;
// Define a method
public void Introduce()
{
Console.WriteLine("My name is " + FirstName + " " + LastName);
}
}
Create an object called person under Person class and initialize it with some values.
var person = new Person();
person.FirstName = "Doris";
person.LastName = "Siu";
person.Introduce();
Encapsulation
Encapsulation is the OOP principle that data is hidden from random access by outside methods of other classes. In line with this principle, the implementation details which refer to the data are declared as private, and methods are declared as public.
You might wonder what means “private” and “public” here, they are actually the access modifiers that restrict the visibility of the members of a class. A public modifier provides the highest level of accessibility in which class, field or method is accessible throughout from within or outside the class.
Meanwhile, private modifier provides the lowest level of accessibility in which a class, field or method is not accessible outside the class.
public class Person
{
// this field is declared as private. The name of the variable needs to be prefixed with “_” to indicate it is a private field by convention.
private DateTime _birthdate;
// provide getter and setter methods as public
public void SetBirthdate(DateTime birthdate)
{
_birthdate = birthdate;
}
public DateTime GetBirthdate()
{
return _birthdate;
}
}
Inheritance
Inheritance is the ability of one class to inherit the members including properties and methods of another class. This allows for code reuse and makes it easier to create complex objects.
The Base Class (aka Parent Class) is a class, from which other classes are inherited while the Derived Class (aka Child Class), is a class that is created from an existing Base Class.
The simplest type of inheritance is single-level inheritance in which a class inherits properties and methods from only one class. Simply speaking, there is only one base class and only one derived class.
The Base Class:
public class PresentationObject
{
public int Width { get; set; }
public int Height { get; set; }
public void Copy()
{
Console.WriteLine("Object copied to clipboard.");
}
public void Duplicate()
{
Console.WriteLine("Object was duplicated.");
}
}
The Derived Class Text:
// the syntax to indicate the inheritance relationship
public class Text : PresentationObject
{
public int FontSize { get; set; }
public string FontName { get; set; }
public void AddHyperlink (string url)
{
Console.WriteLine("We added a link to " + url);
}
}